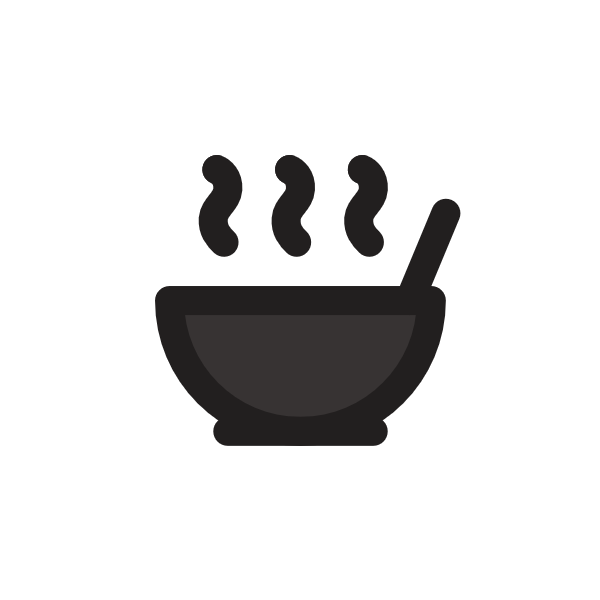
Cocojunk
🚀 Dive deep with CocoJunk – your destination for detailed, well-researched articles across science, technology, culture, and more. Explore knowledge that matters, explained in plain English.
Computer architecture
Read the original article here.
Understanding Computer Architecture: A Foundation for Building from Scratch
Building a computer from scratch is an ambitious project that requires understanding not just how to wire components together, but why those components are designed and interact in specific ways. At the heart of this understanding lies Computer Architecture. Think of computer architecture as the blueprint and philosophy behind how a computer system is designed and how its various parts work together. It's the fundamental structure that dictates everything from the instructions the processor can understand to how fast it can execute them and how it manages memory.
Computer Architecture: A description of the structure of a computer system made from component parts. It encompasses the design philosophy and structure, defining how the hardware and software interact at a fundamental level.
For someone embarking on the journey of building a computer from the ground up, understanding computer architecture is essential. It helps you make informed decisions about design choices, debug issues, and appreciate the complexity and ingenuity involved in creating even the simplest functional computer.
A Brief Look Back: The Evolution of Architectural Ideas
The concept of computer architecture has evolved significantly since the earliest mechanical and electromechanical calculators. The foundational ideas were laid long before the term "computer architecture" was coined.
- Early Concepts: Thinkers like Charles Babbage and Ada Lovelace described the Analytical Engine, a mechanical computer concept, detailing its structure and operations in a way that foreshadowed modern architecture. Konrad Zuse, in designing his Z1 computer in the 1930s, conceived of storing program instructions and data in the same memory space – a revolutionary idea known as the stored-program concept.
Stored-Program Concept: The idea that computer instructions, like data, can be stored in the computer's main memory. This allows for greater flexibility, as the computer can execute different programs simply by loading them into memory, rather than requiring physical rewiring or setup for each task.
- Formalizing the Structure: Pivotal contributions came in the 1940s with papers like John von Neumann's "First Draft of a Report on the EDVAC" and Alan Turing's "Proposed Electronic Calculator" (for the ACE). These documents described the logical organization of electronic computers, formalizing many principles still used today, including the stored-program model often referred to as the "von Neumann architecture."
- The Term "Architecture": The term "architecture" in the context of computers was popularized by Lyle R. Johnson and Frederick P. Brooks, Jr. at IBM in the late 1950s. They used it to describe the level of detail needed to define the system's capabilities from a user's or programmer's perspective, distinct from the underlying hardware implementation details. This concept was famously applied to the IBM System/360, where a consistent "architecture" allowed multiple models with different performance and cost points to run the same software.
- Evolution of Design Methods: Initially, computer architectures were designed on paper and built directly. As complexity grew, prototyping became necessary. Early prototypes used technologies like Transistor-Transistor Logic (TTL) – building blocks of digital circuits. Today, architects commonly use sophisticated computer architecture simulators running on existing computers or implement designs on Field-Programmable Gate Arrays (FPGAs) to test and refine the architecture before committing to costly integrated circuit (IC) manufacturing. This iterative testing and tweaking process is vital. For hobbyist builders, FPGAs often represent a practical platform for implementing and testing custom architectures.
The Layers of Computer Architecture
Computer architecture isn't a single concept but is often discussed in terms of distinct layers or subcategories, moving from the abstract definition of what the machine can do down to the physical wiring. Understanding these layers is crucial for building or even just comprehending how a computer works.
The main subcategories typically discussed are:
- Instruction Set Architecture (ISA): This is the most abstract layer, defining what the computer can do from a programmer's perspective.
- Microarchitecture: This layer describes how the ISA is implemented within a specific processor design.
- Systems Design: This encompasses the architecture of the entire computer system, including components beyond the CPU.
Let's explore these in detail, along with other related concepts.
1. Instruction Set Architecture (ISA)
The ISA is perhaps the most fundamental concept for someone interacting directly with the machine at a low level, as you would when building one.
Instruction Set Architecture (ISA): Defines the set of instructions that a processor can execute, along with the data types, registers, addressing modes, and memory organization accessible to a programmer or software. It is the interface between hardware and software.
Imagine you are building a simple calculator. The ISA would define the buttons it has (+
, -
, *
, /
, =
, Clear
), how you input numbers, how you store intermediate results (maybe one memory slot), and how you tell it to perform an operation (Enter 5
, +
, Enter 3
, =
).
In a computer, the ISA defines:
- Instructions: The specific operations the CPU can perform (e.g., add two numbers, move data from memory to a register, jump to a different part of the program). These instructions are represented numerically, typically in binary.
- Data Types: The types of data the instructions can operate on (e.g., integers of specific sizes, floating-point numbers).
- Registers: A small, fast set of storage locations directly within the CPU that can be accessed quickly by instructions. The ISA defines how many registers there are and how they are referred to (e.g., R0, R1, etc.).
- Addressing Modes: How instructions specify the location of the data they need (e.g., data directly in the instruction, data in a register, data in memory at an address stored in a register).
Register: A small, high-speed storage location within the CPU used to hold data or control information (like the address of the next instruction) that the CPU is currently working with. They are the fastest form of memory accessible to the CPU's execution units.
Addressing Mode: A method used by instructions to calculate or determine the actual memory address of the data operand. Examples include immediate addressing (data is part of the instruction), register addressing (data is in a register), direct addressing (address is part of the instruction), and indirect addressing (address is stored in a register or memory location).
Since processors only understand these binary instructions, software development tools are needed to translate human-readable code into this machine code:
- Compilers: Translate high-level programming languages (like C, C++, Java) into machine code specific to a target ISA.
- Assemblers: Translate human-readable mnemonics (short, symbolic names for instructions, like
ADD
,MOV
) written in assembly language into their corresponding binary machine code representations for a specific ISA. This is often the lowest level a human programmer interacts with directly.
Assembler: A program that translates assembly language source code into machine code executable by a specific processor architecture. Assembly language uses mnemonic codes for instructions and symbolic names for memory locations and registers, making it more readable than raw binary.
Disassembler: A program that performs the reverse process of an assembler, translating machine code back into assembly language. This is often used in debugging or reverse engineering to understand the instructions a program is executing.
The quality and complexity of an ISA involve trade-offs. A complex ISA might allow a single instruction to do a lot, potentially leading to smaller programs ("code size") but requiring more complex hardware to decode and execute the instruction, potentially slowing down the processor or making it more expensive/power-hungry. A simpler ISA (like RISC - Reduced Instruction Set Computing) might require more instructions to achieve the same task, increasing code size, but the instructions are faster and simpler to execute, potentially leading to faster overall performance and simpler hardware.
2. Microarchitecture (Computer Organization)
While the ISA defines what the processor does, the microarchitecture defines how it does it.
Microarchitecture (or Computer Organization): The design of a processor's internal structure to implement a specific Instruction Set Architecture (ISA). It details the data paths, execution units, memory hierarchy (like caches), pipelining strategies, and other internal components and their connections.
Think back to the calculator analogy. If the ISA is the set of buttons and basic operations, the microarchitecture is the internal wiring, the gears and levers (or circuits), how numbers are temporarily held and moved around inside, and the specific sequence of steps taken when you press the '+' button.
Microarchitectural details are typically hidden from the programmer writing code at the ISA level. For example, whether a processor has a large or small CPU cache doesn't change the instructions you write (the ISA), but it significantly impacts the processor's performance (the microarchitecture).
CPU Cache: A small, high-speed memory located near or on the CPU itself, used to store copies of frequently accessed data and instructions from main memory. Accessing data from the cache is much faster than accessing it from main memory, improving performance. Cache size and organization are microarchitectural decisions.
Pipeline: A technique used in microarchitecture to allow multiple instructions to be processed simultaneously at different stages of execution (like fetching, decoding, executing). This increases the throughput of the processor, although it can increase the time taken for a single instruction to complete (latency).
Microarchitecture design is crucial for optimizing performance, power efficiency, and cost. For a builder, understanding microarchitecture helps in:
- Component Selection: Choosing a processor with a suitable microarchitecture for the intended tasks (e.g., needing specific floating-point units for scientific computing, needing fast interrupt handling for real-time systems).
- System Planning: Deciding on other components that complement the CPU's microarchitecture, such as the memory subsystem (including cache considerations, types of RAM) or peripherals (like DMA controllers for efficient data transfer without constant CPU involvement).
- Performance Tuning: Understanding how the internal structure affects execution speed allows for optimizing software or hardware to better utilize the processor's capabilities.
3. Implementation
Implementation is the process of taking the designed architecture (both ISA and microarchitecture) and turning it into physical hardware.
Implementation: The process of translating the architectural design (ISA and microarchitecture) into a physical hardware realization. This involves designing and manufacturing the actual electronic circuits.
This stage involves several steps:
- Logic Implementation: Designing the circuits using basic logic gates (AND, OR, NOT, etc.) to perform the operations defined by the microarchitecture. This is often done using Hardware Description Languages (HDLs) like Verilog or VHDL.
Logic Gate: A basic building block of digital circuits that performs a simple logical operation (like AND, OR, NOT) on one or more binary inputs to produce a single binary output. Complex digital systems are built from millions or billions of interconnected logic gates.
- Circuit Implementation: Designing the actual electronic circuits at the transistor level. This involves deciding how transistors will be arranged to form the logic gates and larger functional blocks (like arithmetic logic units or cache memory).
Transistor: A semiconductor device that acts as a switch or amplifier. It is the fundamental building block of modern integrated circuits, including CPUs and memory.
Integrated Circuit (IC) / Chip: A miniature electronic circuit consisting of many interconnected transistors and other components fabricated on a single piece of semiconductor material (typically silicon).
- Physical Implementation: The layout of the circuit components (transistors, gates, wires) onto a chip (for integrated circuits) or a circuit board. This involves placing components and routing the connections between them, considering factors like signal integrity, power distribution, and heat dissipation.
- Design Validation: Rigorously testing the implemented design to ensure it functions correctly under all conditions and timing constraints before manufacturing. This often involves simulations, logic emulators, and building prototypes.
For a "from scratch" builder using modern techniques like FPGAs, the logic and circuit implementation stages often involve writing HDL code, and the physical implementation is handled by the FPGA manufacturer's tools (mapping the design onto the FPGA's internal structure). Building an actual Integrated Circuit from scratch is significantly more complex and cost-prohibitive for most hobbyists, but understanding this process highlights the journey from abstract idea to physical reality.
Field-Programmable Gate Array (FPGA): An integrated circuit designed to be configured by a customer or designer after manufacturing. FPGAs contain arrays of programmable logic blocks and reconfigurable interconnects, allowing them to implement custom digital circuits, including entire CPU designs (often called "soft microprocessors"). They are commonly used for prototyping and custom hardware.
Other Architectural Concepts
Beyond the main three, other layers and concepts are sometimes discussed:
- Macroarchitecture: Layers of abstraction above the microarchitecture, often related to software interfaces or specific instruction set variations handled by tools.
- Microcode: A layer of software/firmware that translates ISA instructions into a sequence of lower-level micro-operations that the specific hardware (microarchitecture) can execute. This allows chip designers flexibility, such as enabling different processors to implement the same ISA (for software compatibility) or allowing one piece of hardware to emulate multiple ISAs.
- Pin Architecture: Defines the signals and functions available on the external pins of a processor chip, essential for connecting the processor to other components on a circuit board (memory, peripherals, etc.). For a builder wiring up a CPU, understanding the pin architecture is absolutely critical.
Design Goals and Considerations
When designing a computer architecture, engineers must balance many competing goals based on the intended use case. There is no single "best" architecture; the optimal design depends on what you want the computer to do. Common considerations include:
- Performance: How fast can the computer execute tasks?
- Power Efficiency: How much power does the computer consume relative to its performance?
- Cost: How expensive is it to design and manufacture?
- Reliability: How likely is the system to operate without errors?
- Latency vs. Throughput: Are quick responses to single events more important, or is processing a large volume of work per unit time the priority?
- Size, Weight, and Other Factors: Especially important for mobile or embedded systems.
Performance
Measuring performance is complex. Early computers were often compared by clock speed (cycles per second, e.g., MHz or GHz), but this is misleading. A higher clock speed doesn't necessarily mean a faster computer if it takes many clock cycles to complete one instruction.
A better metric is Instructions Per Cycle (IPC).
Instructions Per Cycle (IPC): A measure of how many instructions a processor can execute, on average, in a single clock cycle. A higher IPC indicates greater efficiency in the processor's microarchitecture.
Modern processors can execute multiple instructions per cycle (superscalar processors), achieving IPCs greater than 1. Comparing IPC across different ISAs can be tricky, as what constitutes an "instruction" can vary in complexity.
Another key distinction in performance is between Latency and Throughput:
Latency: The time delay between initiating an operation (like requesting data from memory or sending an instruction) and its completion or the start of the response. It's the time taken for a single event.
Throughput: The total amount of work performed or data processed per unit of time (e.g., instructions per second, data transferred per second). It's a measure of the rate of work.
Often, architectural choices involve trading these off. For example, pipelining (a microarchitectural technique) increases throughput by overlapping instruction execution but can increase the latency of any single instruction due to the stages it must pass through.
For systems that interact with the physical world in real-time (like control systems or anti-lock brakes), interrupt latency (the time between an external event occurring and the system beginning to respond) is often a critical design goal. Failure to respond within a guaranteed time window can lead to system failure.
Benchmarking is used to estimate real-world performance by running standardized test programs. However, benchmarks have limitations, as architectures can be optimized for specific types of tasks or even specific benchmarks, and performance varies significantly depending on the actual software being run.
Power Efficiency
With the rise of mobile computing and concerns about energy consumption, power efficiency has become a primary design goal.
Power Efficiency: The performance achieved per unit of power consumed. A common metric in computer architecture is MIPS/W (Millions of Instructions Per Second per Watt), although more specific benchmarks exist.
Historically, designers focused on putting more and more transistors on a chip (following Moore's Law) and increasing clock speed. As the benefits of simply increasing clock speed have diminished (due to heat and power limits), and the rate of shrinking transistors has slowed, improving the amount of work done per watt has become increasingly important. Modern microarchitectures often include sophisticated power management features. This is particularly critical for battery-powered devices or large data centers where electricity costs are significant.
Recent shifts in market demand, particularly towards mobile and embedded devices, have reinforced this focus on power efficiency and miniaturization over raw clock speed increases, as seen in the design priorities of modern processor generations.
Conclusion
Computer architecture provides the essential framework for understanding how computers are designed, from the fundamental instructions they execute down to the physical arrangement of transistors. For anyone venturing into the "lost art of building a computer from scratch," grasping these concepts – the ISA, microarchitecture, implementation process, and the trade-offs involved in design goals like performance and power efficiency – is not just academic. It's the knowledge that empowers you to design your own simple processor, select appropriate components, understand datasheets, write low-level code, and debug the complex interactions of a working computer system. It turns the task from simply assembling parts according to instructions into a journey of true understanding and creation.